Actions Ad Unit
Actions Ad Unit
Appnext Actions is a non-ad product that enables app developers to turn their app into a platform by enabling seamless integration of third-party services.
By connecting users to other apps and websites from within their apps, publishers are able to increase time spent with their app and generate a new revenue stream that complements their existing ad-monetization strategies.
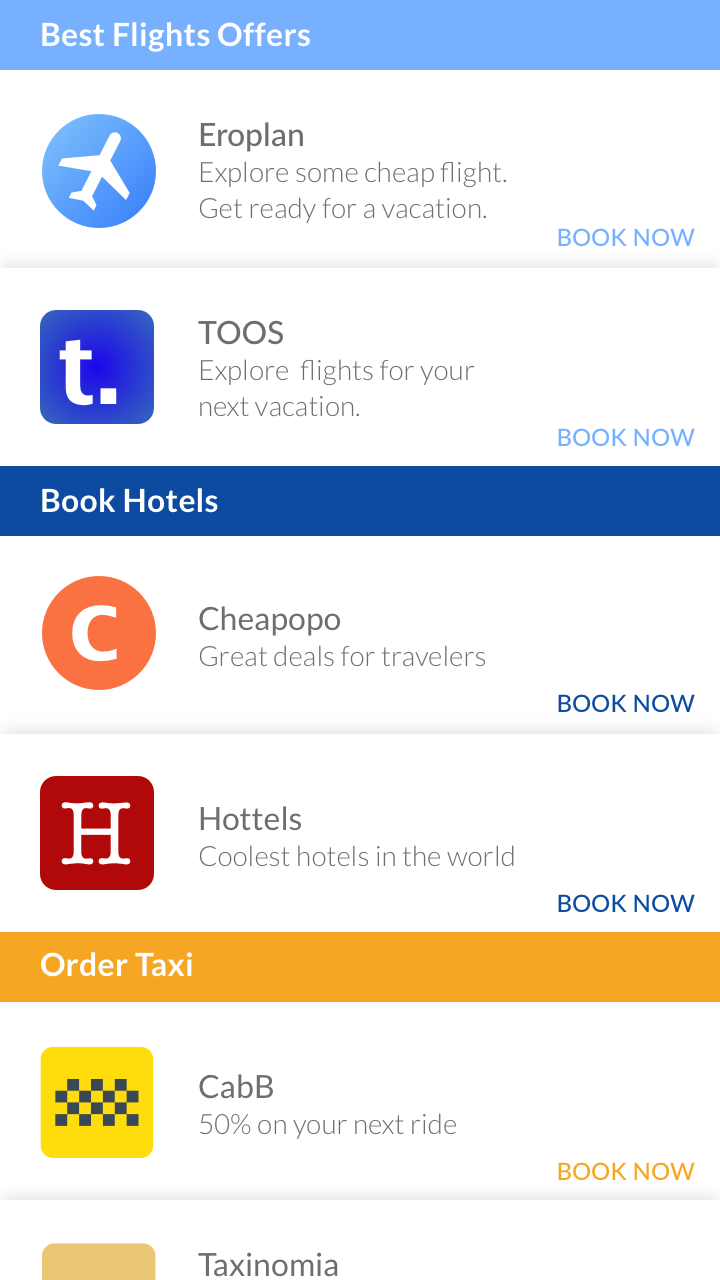
Appnext Actions can be integrated with various apps including Web browsers, Text messaging, Keyboards, Lock screens, and enables publishers to display many actions such as order food, shop, book a hotel, listen to music, order a taxi, watch a movie and more.
1. Action Display
Actions SDK provides you with the action data (icon & name) allowing you to design your own customized UI display for Actions in your app.
Actions can be displayed based on your specific selection in the code, or on user intent (moment based logic).
2. Actions Selection
Once a user click on an action, he/she is provided with an Android engaging native UI with various apps available for completing the action.
3. Service selection
A user can will be able to choose which app to use. Once the service is clicked:
~ If the service is an app : the app will open (if already installed) or will redirect to the play store for download.
~ If the service is a website : the user will be redirected to the website.
Actions can be integrated in any app by using the Actions SDK. Full documentation provided below.
Suggesting a specific action based on user keywords (which are are action and specific and customized per publisher based on cultural and additional aspects). The suggestion could appear on the first option of the auto complete list (browser), as buttons above the input textbox or as yes/no friendly questions (messengers & keyboards).
Action can be a big advantage for lockscreen apps - using the moment based action you can always get to your users suggeting them apps through their daily moments.
Suggested to implement action buttons to display the user moment based actions or specific content related action.
Integrate buttons of moment based actions or display a random action, between game levels of or in menu pages.
Latest version: 2.4.2.471.2 (released on August 1st, 2018)
SDK Installation Notes:
- Integrate the latest version of Google Play Services, in your app. Instructions can be found here
- The Android Actions SDK is supported on OS version 4.2 and above
- Any combination of the ad units should reflect adding the relevant line to the module gradle.
Google Play Services:
In order to use the Actions SDK it is required to integrate the latest version of Google Play Services in your app. In case you do not wish to integrate all Google Play Services APIs, make sure to include the following APIs:
- Google Actions, Base Client Library - basement
- Google Location and Activity Recognition
App Permissions
The Appnext SDK uses the permissions granted to your app in order to improve campaigns targeting, and in order to suggest the most relevant apps to your users.
We highly recommend that your app request the following permissions so we can better suggest apps to your users, and serve premium campaign types in your app.
Make the following changes in AndroidManifest.xml of your project :
android.gms.permission.ACTIVITY_RECOGNITION
android.permission.ACCESS_WIFI_STATE
android.permission.CHANGE_WIFI_STATE
android.permission.READ_PHONE_STATE
android.permission.ACCESS_FINE_LOCATION
android.permission.ACCESS_COARSE_LOCATION
android.permission.GET_TASKS
android.permission.REAL_GET_TASKS
android.permission.PACKAGE_USAGE_STATS
android.permission.WRITE_EXTERNAL_STORAGE
android.permission.ACCESS_NETWORK_STATE
android.permission.INTERNET
android.permission.BLUETOOTH_ADMIN
Integrate Appnext Actions SDK using gradle
In order to integrate Actions latest SDK, simply add the following to your appโs build.gradle file inside repositories section.
allprojects {
repositories {
maven { url "http://dl.appnext.com/" }
}
}
Then add the following to the dependencies section:
dependencies {
implementation 'com.appnext.sdk:actions:+'
implementation 'com.google.android.gms:play-services-basement:11.0.0' // Mandatory
implementation 'com.google.android.gms:play-services-location:11.0.0' // Mandatory
}
Step 1:
Step 2: Import References
import com.appnext.actionssdk.ActionSDK;
Step 3: Initialization
Initialize the Actions SDK using the activity and your app's Placement ID in the constructor.
ActionSDK actionSDK = new ActionSDK(getActivity(), "<PlacementID>");
Step 4: Load Actions
Loading the actions - 3 options:
A - Specific Actions - get a specific single action or a list of specific actions
B - All moment based Actions - get Actions (once) based on the user's current moment
C - Register for moment based Actions - subscribe to get Actions each whenever a user's moment is detected.
A - Load a specific Action
loadActions
retrieves specific actions for display, passing the action params as enum values. See the full params actions list.
actionSDK.loadActions(new OnActionsLoaded() {
@Override
public void onActionsLoaded(ArrayList<ActionData> actions) {
// Place your code here
}
}, Action.ORDER_A_TAXI, Action.ORDER_FOOD, ...); //*"action1", "action2", ...*/
B - Load all moment based Actions
Requests actions based on user moment.
loadMoments
Gets the onActionsLoaded
callback, retrieves ActionData
objects and displays it.
actionSDK.loadMoments(new OnActionsLoaded() {
@Override
public void onActionsLoaded(ArrayList<ActionData> actions) {
// Place your code here
}
});
C - Load Actions by registering for moment based Actions
The moments registration method notifies whenever relevant (moment based) action/s are available, and then displays it. startMomentCallback
gets the onRecieve
callback, retrieves ActionData
objects and displays it.
@Override
public void onResume() {
super.onResume();
actionSDK.startMomentCallback(new ActionSDK.MomentsCallback() {
@Override
public void onReceive(ArrayList<ActionData> actions) {
// Place you code here
}
});
}
@Override
public void onPause() {
super.onPause();
actionSDK.stopMomentCallback();
}
An alternative for checking if an action was loaded
Instead of using the
onActionsLoaded
callback, you can alternatively use theisActionLoaded
method. It expects to get the Action enum ("action param"), and retrieves a boolean that indicates whether a specific action was loaded successfully.
actionSDK.isActionLoaded(Action.ORDER_A_TAXI);
Step 5: Display the Action
Place the below code whenever you want to actually display the Action.
For every actionData
retrieved (in each of the above callbacks onActionsLoaded
onReceive
):
Step B. Display the ActionData
retrieved (your own implementation).
@Override
public void onActionsLoaded(ArrayList<ActionData> actions) {
actionData = actions.get(0);
// step A: check Action expiry
if (!actionData.getExpire())
{
// step B: display the actionData
// place your code here. For example:
// viewHolder.image.setImageDrawable(actionData.getActionIcon(context));
// step C: call actionDisplayed
actionSDK.actionDisplayed(actionData);
}
}
Step 6: Display apps list
On Action click, showAction
should be called in order for the apps list to be displayed. It is a void function that expects to get the clicked action enum.
actionSDK.showAction(Action.ORDER_A_TAXI);
Step 7: ActionData
properties
ActionData
propertiesgetActionParam
getActionParam
An action unique string identifier. see actions-to-params mapping in the table below.
getActionName
getActionName
The action name. see the actions list in the table below.
getExpire
getExpire
The action expiry time (in Unix time).This is the time remained for the Action to be valid for display. After that time expires Action should be requested again, since expiration means that it was loaded but wasn't yet displayed for some time. If the user clicks on an expired action, the SDK will not display anything.
It is mandatory to check the actionโs expiry time and request a new action once the current action is detected as expired.
getActionDynamicMessage
getActionDynamicMessage
An action related message for display on the action button, which consists a meaningful content taken on real time from one of the action related apps (while the displayed data and selection logic are action based). For example, for โOrder a taxiโ action, the customized message can be โYour taxi will arrive in 3 minsโ.
In this action:
a. The ETA is the data being calculated at real time, using userโs current location
b. The message selection logic will be the one suggesting the lowest ETA
Properties Usage:
String actionParam = action.getActionParam();
String actionName = action.getActionName();
String actionExpiry = action.getExpire();
String actionMessage = action.getActionDynamicMessage();
Properties print outs example:
getActionParam: sapat
getActionName: Play an action game
getExpire: 1485347337
getActionDynamicMessage: Your Taxi will arrive in 4 mins
Step 8: Set icons color
Set all the actions icons color to black or white.
actionSDK.setActionIconColor("white");
actionSDK.setActionIconColor("black");
Step 9: Destroy
onDestroy
is a void function that should to be called when your app is closed.
actionSDK.onDestroy();
Step 10: Event callbacks registration (optional)
In order to receive client-side callbacks, please import the following references:
import com.appnext.actionssdk.callback.OnActionOpened;
import com.appnext.actionssdk.callback.OnAppClicked;
import com.appnext.actionssdk.callback.OnActionClosed;
import com.appnext.actionssdk.callback.OnActionError;
// Receive an event callback for action opened.
// Fired when *apps list is displayed*.
actionSDK.setOnActionOpenedCallback(new OnActionOpened() {
@Override
public void actionOpened() {
Log.v("action", "opened");
}
});
// Receive an event callback for app clicked.
// Fired on *app click* in the apps list.
actionSDK.setOnAppClickedCallback(new OnAppClicked() {
@Override
public void appClicked() {
Log.v("app", "clicked");
}
});
// Receive an event callback when the apps list is closed.
// Fired whenever the user *closed the apps list*.
actionSDK.setOnActionClosedCallback(new OnActionClosed() {
@Override
public void actionClosed() {
Log.v("action", "closed");
}
});
// Receive an event callback for Action error (including the action name).
// Fired whenever *error is detected*.
// Possible errors:
- `NO_ADS`
- `CONNECTION_ERROR`
- `SLOW_CONNECTION`
- `INTERNAL_ERROR`
- `NO_MARKET`
- `ACTION_NOT_READY`
- `ACTION_EXPIRED`
actionSDK.setOnActionErrorCallback(new OnActionError() {
@Override
public void actionError(String action, String error) {
Log.v("action", "action: " + action + " error: " + error);
}
});
Actions list
Please click here to see the list of all available Actions and their parameters.
Integration Support
Good luck integrating! Please feel free to ask us any question at Appnext support.
Updated over 5 years ago