Rewarded and Fullscreen Video
Appnext Android SDK - Rewarded and Fullscreen video ad unit
Ad unit Integration
Step 1 – Initialize the SDK
Initialize the SDK whenever your app is loaded. Add the following code to your app's main activity:
Appnext.init(context);
Step 2: Define a new Ad
Make sure to set your app's in the constructor of this class.
//Fullscreen
FullScreenVideo fullscreen_ad = new FullScreenVideo(this, ADD_HERE_YOUR_PLACEMENT_ID);
Or
//Rewarded
RewardedVideo rewarded_ad = new RewardedVideo(this, ADD_HERE_YOUR_PLACEMENT_ID);
New! Get the predicted ECPM value before loading the ad. Check out Appnext solution for in-app header bidding here.
Step 3: Loading an Ad
//Fullscreen
fullscreen_ad.loadAd();
Or
//Rewarded
rewarded_ad.loadAd();
Notes:
- You must load the ad before showing it. In case the loadAd function was not called, the SDK will return an error - "ad not ready"
- It may take time for an ad to load and be ready to show, depending on the user's internet speed. Make sure to load the ad as earlier as possible
Step 4: Showing the Ad
//Fullscreen
if (fullscreen_ad.isAdLoaded()){
fullscreen_ad.showAd();
}else{
//continue...
}
Or
//Rewarded
if (rewarded_ad.isAdLoaded()){
rewarded_ad.showAd();
}else{
//continue...
}
Step 5 (optional): Callbacks
In order to receive client-side callbacks, import the following references:
import com.appnext.core.callbacks.OnAdLoaded;
import com.appnext.core.callbacks.OnAdOpened;
import com.appnext.core.callbacks.OnAdClicked;
import com.appnext.core.callbacks.OnAdClosed;
import com.appnext.core.callbacks.OnAdError;
import com.appnext.core.callbacks.OnVideoEnded;
Receive event callbacks from the SDK:
Fullscreen:
// Get callback for ad loaded
fullscreen.setOnAdLoadedCallback(new OnAdLoaded() {
@Override
public void adLoaded(String bannerID,AppnextAdCreativeType creativeType) {
}
});
// Get callback for ad opened
fullscreen.setOnAdOpenedCallback(new OnAdOpened() {
@Override
public void adOpened() {
}
});
// Get callback for ad clicked
fullscreen.setOnAdClickedCallback(new OnAdClicked() {
@Override
public void adClicked() {
}
});
// Get callback for ad closed
fullscreen.setOnAdClosedCallback(new OnAdClosed() {
@Override
public void onAdClosed() {
}
});
// Get callback for ad error
fullscreen.setOnAdErrorCallback(new OnAdError() {
@Override
public void adError(String error) {
}
});
// Get callback when the user saw the video until the end (video ended)
fullscreen.setOnVideoEndedCallback(new OnVideoEnded() {
@Override
public void videoEnded() {
}
});
Rewarded:
// Get callback for ad loaded
rewarded_ad.setOnAdLoadedCallback(new OnAdLoaded() {
@Override
public void adLoaded(String bannerID) {
}
});
// Get callback for ad opened
rewarded_ad.setOnAdOpenedCallback(new OnAdOpened() {
@Override
public void adOpened() {
}
});
// Get callback for ad clicked
rewarded_ad.setOnAdClickedCallback(new OnAdClicked() {
@Override
public void adClicked() {
}
});
// Get callback for ad closed
rewarded_ad.setOnAdClosedCallback(new OnAdClosed() {
@Override
public void onAdClosed() {
}
});
// Get callback for ad error
rewarded_ad.setOnAdErrorCallback(new OnAdError() {
@Override
public void adError(String error) {
}
});
// Get callback when the user saw the video until the end (video ended)
rewarded_ad.setOnVideoEndedCallback(new OnVideoEnded() {
@Override
public void videoEnded() {
}
});
The possible errors we pass in the adError callback are (AdsError):
- CONNECTION_ERROR
- SLOW_CONNECTION
- AD_NOT_READY
- NO_ADS
- INTERNAL_ERROR
- NO_MARKET
- TIMEOUT
Example for rewarded ad:
rewarded_ad.setOnAdErrorCallback(new OnAdError() {
@Override
public void adError(String error) {
switch (error){
case AdsError.NO_ADS:
Log.v("appnext", "no ads");
break;
case AdsError.CONNECTION_ERROR:
Log.v("appnext", "connection problem");
break;
default:
Log.v("appnext", "other error");
}
}
});
Example for fullscreen ad:
fullscreen_ad.setOnAdErrorCallback(new OnAdError() {
@Override
public void adError(String error) {
switch (error){
case AppnextError.NO_ADS:
Log.v("appnext", "no ads");
break;
case AppnextError.CONNECTION_ERROR:
Log.v("appnext", "connection problem");
break;
default:
Log.v("appnext", "other error");
}
}
});
Ad unit UX
Rewarded Video ad unit
The Rewarded ad unit includes three screens by default; pre-roll screen, roll screen and the post-roll screen.
- The pre-roll screen offers the user an option to choose the rewarded video (configurable using the
setMode
setter). The user can click the right or the left video thumbnails. Clicking a thumbnail will start play the video. The selection is limited in time (configurable using thesetMultiTimerLength
setter). If no selection is made, a random selection of the video is made by the ad unit. - The roll screen is the screen that the rewarded video plays. In this screen, the user can click the call-to-action button. Clicking it once will change the button into a "V" icon, clicking it more will show a caption to the user, that a redirection to the store will happen once the video is ended. The caption is shown for 3 seconds by default (configurable using the
setRollCaptionTime
). - The post-roll screen is shown to the user once the video is ended. The user can close the ad, or choose to install the advertised app or other suggested apps.
Viewing the rewarded video
- When using the rewarded video the user must see the entire video
- When "normal" mode is used, only the roll and post-roll screens will be shown to the user
- Clicking the "i" icon on the roll screen will show Appnext privacy policy landing page
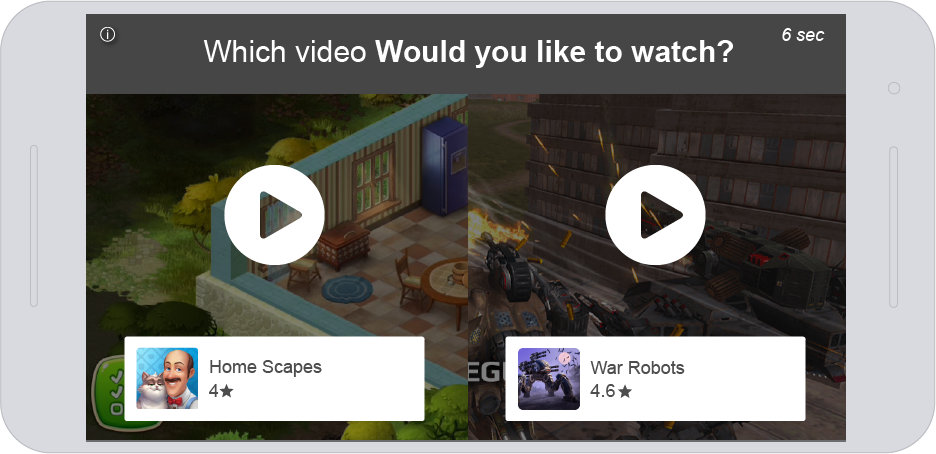
The pre-roll screen with the video selection
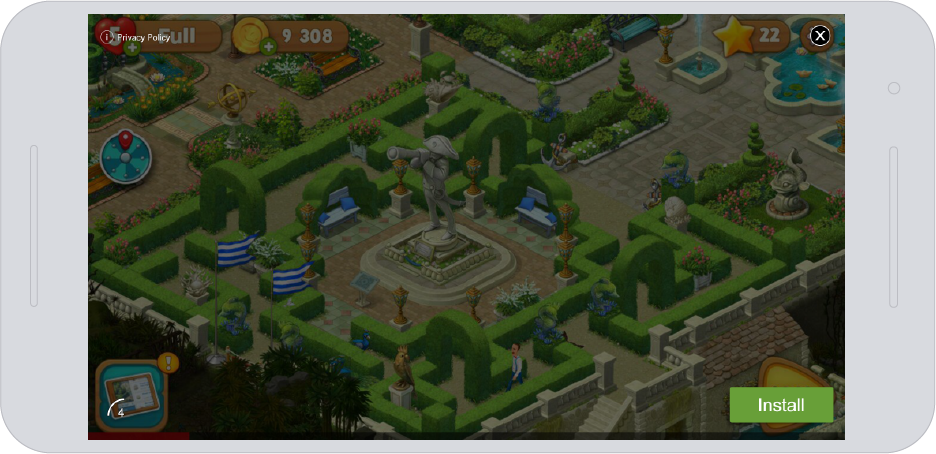
The roll screen while playing
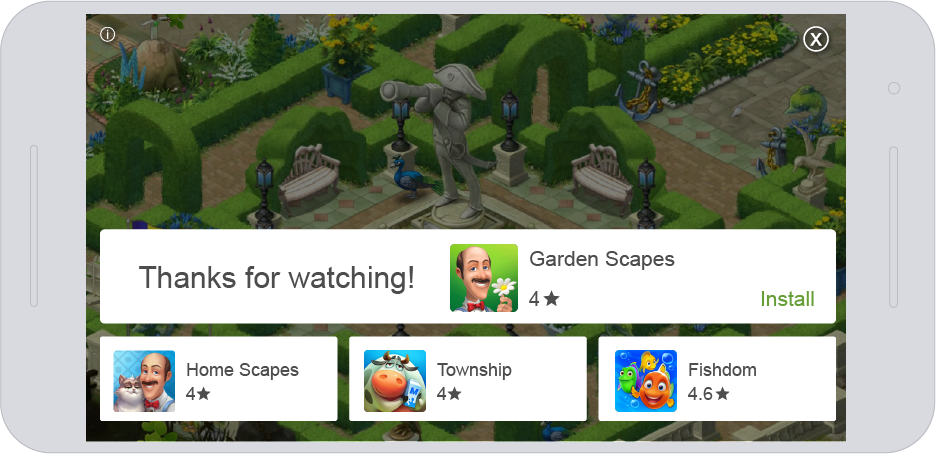
The post-roll screen
Fullscreen Video ad unit
The Fullscreen ad unit includes two screens; roll screen and post-roll scren
- The roll screen - The roll screen is the screen that the fullscreen video plays. In this screen, the user can click the call-to-action button. Clicking the call-to-action button will launch the store (without waiting for the video to end). The user can also close the ad by clicking the "X" button. It is possible to delay the "X" button from showing (using the
setShowClose
setter) - The post-roll screen is shown to the user once the video is ended. The user can close the ad, or choose to install the advertised app or other suggested apps.
- Clicking the "i" icon on the roll screen will show Appnext privacy policy landing page
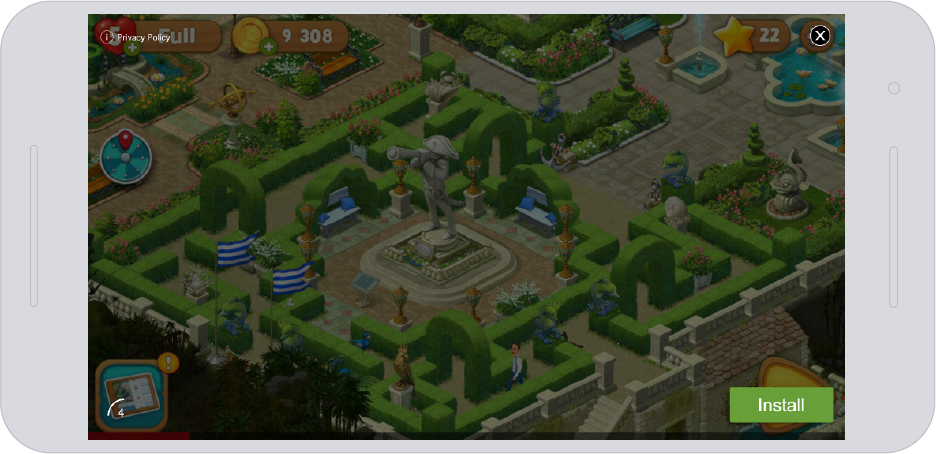
The Fullscreen video roll-screen.
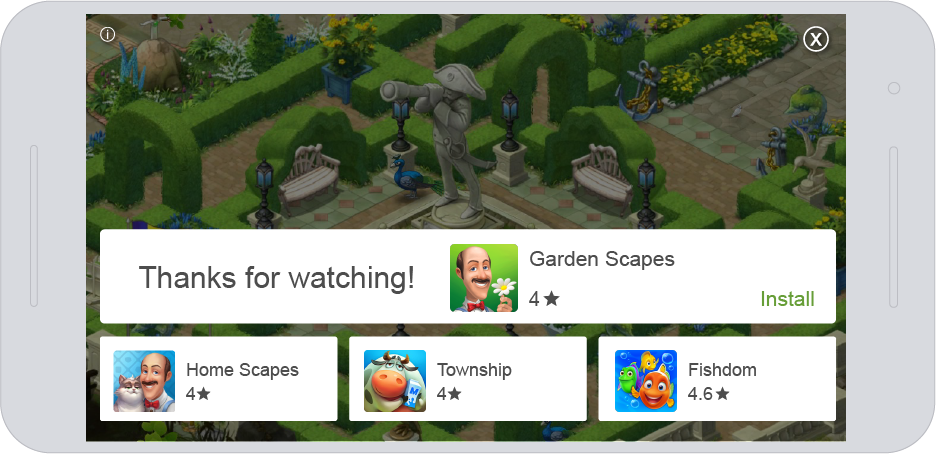
The Fullscreen video post-roll screen
Advanced Settings
Custom Configuration
By default, the SDK configuration will be loaded from Appnext's server. You can change the configuration directly by config or through the setter function.
Using Config
Change to each configuration (Fullscreen / Rewarded) should be made separately.
In order to use custom configuration, import the following class:
//Fullscreen
import com.appnext.ads.fullscreen.FullscreenConfig;
//Rewarded
import com.appnext.ads.fullscreen.RewardedConfig;
Define the ad unit's configuration instance:
//Fullscreen
FullscreenConfig config = new FullscreenConfig();
//Rewarded
RewardedConfig config = new RewardedConfig();
Examples:
//Fullscreen
FullscreenConfig config = new FullscreenConfig();
//or Rewarded
RewardedConfig config = new RewardedConfig();config.setMode(RewardedVideo.VIDEO_MODE_MULTI);
config.setMultiTimerLength(7);
config.setRollCaptionTime(-1);
config.setCategories("category1,category2");
config.setSpecificCategories("category1,category2");
config.setPostback("postback");
config.setMute(false);
config.setVideoLength(Video.VIDEO_LENGTH_DEFAULT);
config.setOrientation(Video.ORIENTATION_DEFAULT);
config.setShowClose(true);
// you can alternatively set the property directly:
// config.categories = "category1,category2";
// config.postback = "postback";
// config.mute = false;
// config.videoLength = FullScreenVideo.VIDEO_LENGTH_DEFAULT;
// config.orientation = FullScreenVideo.ORIENTATION_DEFAULT;
// ...
FullescreenVideo fullscreen_ad = new FullescreenVideo(this, placementID, config);
//or Rewarded
RewardedVideo rewarded_ad = new RewardedVideo(this, placementID, config);CopyRawCancel
//Fullscreen
FullscreenConfig config = new FullscreenConfig();
Using Setters:
Fullscreen:
FullescreenVideo fullscreen_ad = new FullescreenVideo(this, placementID);
fullscreen_ad.setCategories("category1,category2");
fullscreen_ad.setSpecificCategories("category1,category2");
fullscreen_ad.setPostback("postback");
fullscreen_ad.setMute(false);
fullscreen_ad.setVideoLength(Ad.VIDEO_LENGTH_DEFAULT);
fullscreen_ad.setOrientation(Video.ORIENTATION_DEFAULT);
fullscreen_ad.setBackButtonCanClose(false);
fullscreen_ad.setShowClose(true);
fullscreen_ad.setShowCta(false);
fullscreen_ad.setRollCaptionTime(-1);
Rewarded:
RewardedVideo rewarded_ad = new RewardedVideo(this, placementID);
rewarded_ad.setMode(RewardedVideo.VIDEO_MODE_MULTI);
rewarded_ad.setCategories("category1,category2");
rewarded_ad.setMultiTimerLength(7);
rewarded_ad.setPostback("postback");
rewarded_ad.setMute(false);
rewarded_ad.setVideoLength(Video.VIDEO_LENGTH_DEFAULT);
rewarded_ad.setOrientation(ad.ORIENTATION_DEFAULT);
rewarded_ad.setRollCaptionTime(3);
List of configurable options:
Rewarded video mode setMode
setMode
Set the mode the rewarded ad unit will work in:
multi
- with pre-roll video selection screen (default)normal
- without pre-roll video selection screen
Rewarded video video selection timer setMultiTimerLength
setMultiTimerLength
When "multi" mode is on, this function (setMultiTimerLength) sets the number of seconds to make a video selection. The function can accept values from 1 to 20. The default value is 8 seconds.
Roll screen caption time setRollCaptionTime
setRollCaptionTime
The number of seconds that the google play text caption is showing. The caption is shown to the user after clicking the Call-to-action button. The function (setRollCaptionTime) can accepts values from 1 to 20. The default value is 3 seconds. Entering -1 will make the caption visible until the video finish to play
Category setCategories
setCategories
Set preferred ad categories
Install Postback setPostback
setPostback
Postback parameters that will be posted to your server after user installed an app (make sure to encode the values)
Mute Video setMute
setMute
Mute the video which is played in the ad (the default value is "false")
- true
- false
Fullscreen - Show Close Button setShowClose(true,2000)
setShowClose(true,2000)
Display or hide the "x" (close) button
- true
- false
- time in ms to delay the close button from showing - default value is 0
Video Length setVideoLength
setVideoLength
Set video length - 15 or 30 seconds long (default is VIDEO_LENGTH_DEFAULT)
VIDEO_LENGTH_SHORT
- Up to 15 seconds long videosVIDEO_LENGTH_LONG
- 20-60 seconds long videosVIDEO_LENGTH_DEFAULT
- Appnext's algorithm priority. Any available video campaign will be served
Please Note!
Please note that if no SHORT type length videos are available, long videos will be served
Orientation setOrientation
setOrientation
Set the preferred orientation if both landscape and portrait are supported by the application (default is ORIENTATION_DEFAULT
). The ad unit displayed orientation cannot be changed by the user after the ad unit started.
FullScreenVideo.ORIENTATION_DEFAULT
FullScreenVideo.ORIENTATION_LANDSCAPE
FullScreenVideo.ORIENTATION_PORTRAIT
Server-side Postback - Rewarded Video
You can choose to receive server-side postbacks whenever a user finishes watching a video ("video ended" event).The "video ended" event will serve as a trigger for the reward action.
Please note that in addition to configuring the postback in this integration process, you will also need to set your postback URL on the Appnext Self-Service platform (under the "Apps" page / app "Settings & Placements" / "Advanced Settings').
In order to receive the server-side postback on the "video ended" event, call the following functions before showing the ad:
//Transaction ID - make sure to set a unique transaction ID per ad view
rewarded_ad.setRewardsTransactionId("TransactionId");
//User ID - pass the User ID so you will know which user to reward
rewarded_ad.setRewardsUserId("UserId");
//Currency Type - type of reward (life / credit / points)
rewarded_ad.setRewardsRewardTypeCurrency("TypeCurrency");
//Amount - the amount of currency that was rewarded
rewarded_ad.setRewardsAmountRewarded("Amount");
//Custom Parameters - pass any custom value / data
rewarded_ad.setRewardsCustomParameter("CustomParameter");
- Make sure to encode all values passed in each function.
- Passing value, for at least one parameter, is mandatory, for the server-side reward postback to be sent.
Example Project
You can download an example project (Android Studio) from our Github page here.
App Categories
1. Action 2. Adventure 3. Arcade 4. Arcade & Action 5. Board 6. Books & Reference 7. Brain & Puzzle 8. Business 9. Card 10. Cards & Casino 11. Casino 12. Casual 13. Comics 14. Communications 15. Education 16. Educational 17. Entertainment 18. Family 19. Finance 20. Health & Fitness 21. Libraries & Demo 22. Lifestyle | 23. Live Wallpaper 24. Media & Video 25. Medical 26. Music 27. Music & Audio 28. News & Magazines 29. Personalization 30. Photography 31. Productivity 32. Puzzle 33. Racing 35. Shopping 36. Simulation 37. Social 38. Sports 39. Sports Games 40. Strategy 41. Tools 42. Travel & Local 43. Trivia 44. Weather 45. Word |
Make sure to encode (%20) categories with more than 1 word, example: Travel%20%26%20Local
Integration Support
Should you have any problems integrating the product, log a ticket with us by emailing [email protected]
Updated about 4 years ago