Native Ads
Appnext Android SDK - New Native Ads ad unit
SDK Installation
Make sure to Install the SDK in your Android Studio project as described here
Step 1 – Initialize the SDK
Initialize the SDK whenever your app is loaded. Add the following code to your app's main activity:
Appnext.init(context);
Step 2.1 Request a single or multiple Ads
you can choose between
single request : requesting one native Ad or
multiple requests : return X amount of Ads at the same time. Please replace <NUMBER_OF_REQUESTS> with the numbers of Ads you would like to receive.
Single request:
private NativeAdListenerImpl mNativeAdListener;
@Override
protected void onCreate(Bundle savedInstanceState) {
mNativeAdListener = new NativeAdListenerImpl();
NativeAd nativeAd = new NativeAd(this, "<YOUR_PLACEMENT_ID>");
nativeAd.setAdListener(mNativeAdListener);
nativeAd.loadAd(new NativeAdRequest());
}
private class NativeAdListenerImpl extends NativeAdListener{
@Override
public void onAdLoaded(NativeAd nativeAd, AppnextAdCreativeType creativeType) {
super.onAdLoaded(nativeAd, creativeType);
mNativeAds.add(nativeAd);
mAdapter.notifyDataSetChanged();
}
@Override
public void onAdClicked(NativeAd nativeAd) {
super.onAdClicked(nativeAd);
Log.d("NativeAd Clicking", nativeAd.getAdTitle());
}
@Override
public void onError(NativeAd nativeAd, AppnextError appnextError) {
super.onError(nativeAd, appnextError);
Toast.makeText(NewNativeAdsListViewActivity.this, appnextError.getErrorMessage(), Toast.LENGTH_LONG).show();
}
@Override
public void adImpression(NativeAd nativeAd) {
super.adImpression(nativeAd);
Log.d("NativeAd Impression", nativeAd.getAdTitle());
}
}
Multiple request:
private NativeAdListenerImpl mNativeAdListener;
@Override
protected void onCreate(Bundle savedInstanceState) {
mNativeAdListener = new NativeAdListenerImpl();
AdLoader.load(this, "<YOUR_PLACEMENT_ID>",
new NativeAdRequest(),mNativeAdListener, <NUMBER_OF_REQUESTS>);
}
private class NativeAdListenerImpl extends NativeAdListener{
@Override
public void onAdLoaded(NativeAd nativeAd, AppnextAdCreativeType creativeType) {
super.onAdLoaded(nativeAd, creativeType);
mNativeAds.add(nativeAd);
mAdapter.notifyDataSetChanged();
}
@Override
public void onAdClicked(NativeAd nativeAd) {
super.onAdClicked(nativeAd);
Log.d("NativeAd Clicking", nativeAd.getAdTitle());
}
@Override
public void onError(NativeAd nativeAd, AppnextError appnextError) {
super.onError(nativeAd, appnextError);
Toast.makeText(NewNativeAdsListViewActivity.this, appnextError.getErrorMessage(), Toast.LENGTH_LONG).show();
}
@Override
public void adImpression(NativeAd nativeAd) {
super.adImpression(nativeAd);
Log.d("NativeAd Impression", nativeAd.getAdTitle());
}
}
NOTE :
by typing 10 instead of <NUMBER_OF_REQUESTS>, means we will server 10 or less requests. we might server less requests based on our internal serving logic.
Step 2.2: Prepare Call Backs
Create Appnext nativeAd
object with Placement ID, set the NativeAdListener
, and prepare to call the onAdLoaded
event;
The following callbacks are available after loading the nativeAd:
adImpression
- The native ad was viewed by the user, and an impression was reported to AppnextonAdClicked
- The native ad was clicked by the useronError
- An error has occurred. The following errors codes are passed in the onError callback.
(Using theAppnextError
object);NO_ADS
,AD_NOT_READY
,CONNECTION_ERROR
,TIMEOUT
,NO_MARKET
,INTERNAL
NativeAd nativeAd = new NativeAd(this, "ADD_HERE_YOUR_PLACEMENT_ID");
nativeAd.setAdListener(new NativeAdListener() {
@Override
public void onAdLoaded(NativeAd nativeAd, AppnextAdCreativeType creativeType) {
super.onAdLoaded(nativeAd, creativeType);
}
@Override
public void onAdClicked(NativeAd nativeAd) {
super.onAdClicked(nativeAd);
}
@Override
public void onError(NativeAd nativeAd, AppnextError appnextError) {
super.onError(nativeAd, appnextError);
}
@Override
public void adImpression(NativeAd nativeAd) {
super.adImpression(nativeAd);
}
});
nativeAd.loadAd(new NativeAdRequest()
// optional - config your ad request:
.setPostback("")
.setCategories("")
.setCachingPolicy(NativeAdRequest.CachingPolicy.ALL)
.setCreativeType(NativeAdRequest.CreativeType.ALL)
.setVideoLength(NativeAdRequest.VideoLength.SHORT)
.setVideoQuality(NativeAdRequest.VideoQuality.HIGH));
);
}
We will add some code to the
onAdLoaded
callback and modify theNativeAdRequest
object further down in this documentation
Step 3: Create your Native Ad Layout
You can create your custom view (custom ad design) in a layout .xml, or you can add elements in code:
Please make sure to include the ad layout views under the com.appnext.nativeads.NativeAdView
element.
//Creates the `NativeAdView` programmatically
NativeAdView nativeAdView = new NativeAdView(this);
//Adds any other view below the `NativeAdView`
nativeAdView.addView("YOUR VIEW");
parentView.addView(nativeAdView);
Example:
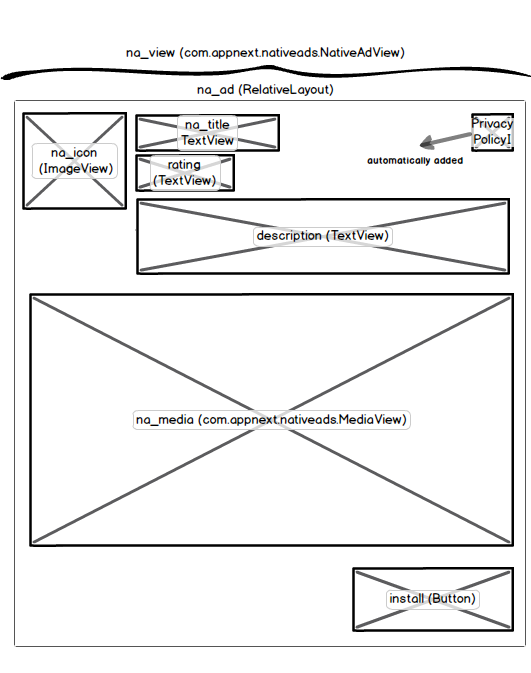
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@android:color/darker_gray"
tools:context="com.example.asaf.appnextnaviveads.InFeedExample1">
<ProgressBar
android:id="@+id/progressBar"
style="?android:attr/progressBarStyle"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true" />
<com.appnext.nativeads.NativeAdView
android:id="@+id/na_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="10dp"
android:layout_marginRight="10dp"
android:layout_marginTop="20dp"
android:background="@android:color/white"
android:padding="10dp">
<RelativeLayout
android:id="@+id/na_ad"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<ImageView
android:id="@+id/na_icon"
android:layout_width="60dp"
android:layout_height="60dp"
android:layout_alignParentStart="true"
android:layout_alignParentTop="true"
android:layout_marginBottom="10dp"
tools:src="@drawable/ic_launcher_foreground" />
<TextView
android:id="@+id/na_title"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_alignParentRight="true"
android:layout_marginLeft="10dp"
android:layout_marginRight="15dp"
android:layout_toRightOf="@id/na_icon"
android:gravity="left"
android:textSize="16sp" />
<TextView
android:id="@+id/rating"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_above="@+id/na_media"
android:layout_below="@+id/na_title"
android:layout_marginLeft="10dp"
android:layout_toEndOf="@+id/na_icon"
android:textSize="16sp" />
<com.appnext.nativeads.MediaView
android:id="@+id/na_media"
android:layout_width="match_parent"
android:layout_height="200dp"
android:layout_below="@id/description" />
<Button
android:id="@+id/install"
android:layout_width="104dp"
android:layout_height="40dp"
android:layout_alignParentEnd="true"
android:layout_below="@+id/na_media"
android:background="@drawable/rounded_button"
android:text="install"
android:textColor="#ffffff" />
<ImageView
android:id="@+id/imageView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/na_title"
android:layout_marginLeft="3dp"
android:layout_marginTop="4dp"
android:layout_toEndOf="@+id/rating"
app:srcCompat="@drawable/apnxt_ads_full_star" />
<TextView
android:id="@+id/description"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignEnd="@+id/na_title"
android:layout_alignStart="@+id/rating"
android:layout_below="@+id/imageView"
android:layout_marginTop="4dp"
tools:text="TextView" />
</RelativeLayout>
</com.appnext.nativeads.NativeAdView>
</RelativeLayout>
Step 4: Inflating the Layout using the nativeAd object
In this step we are inflating the nativeAd
object from step 2, using the dedicated functions and views
Example:
Appnext.init(this);
//Sets the views from the activity layout (step 3)
//see further down in the code
setViews();
NativeAd nativeAd = new NativeAd(this, "ADD_YOUR_PLACEMENT_ID_HERE");
nativeAd.setPrivacyPolicyColor(PrivacyIcon.PP_ICON_COLOR_LIGHT);
nativeAd.setAdListener(new NativeAdListener() {
@Override
public void onAdLoaded(final NativeAd nativeAd) {
super.onAdLoaded(nativeAd);
//The progress bar is optional according to the app UX (not part of Appnext Native Ads ad unit)
progressBar.setVisibility(View.GONE);
//The ad Icon
nativeAd.downloadAndDisplayImage(imageView, nativeAd.getIconURL());
//The ad title
textView.setText(nativeAd.getAdTitle());
//Setting up the Appnext MediaView
nativeAd.setMediaView(mediaView);
//The ad rating
rating.setText(nativeAd.getStoreRating());
//The ad description
description.setText(nativeAd.getAdDescription());
//Registering the clickable areas - see the array object in `setViews()` function
nativeAd.registerClickableViews(nativeAdView);
//Setting up the entire native ad view
nativeAd.setNativeAdView(nativeAdView);
}
//Ad clicked callback
@Override
public void onAdClicked(NativeAd nativeAd) {
super.onAdClicked(nativeAd);
}
//Ad error callback
@Override
public void onError(NativeAd nativeAd, AppnextError appnextError) {
super.onError(nativeAd, appnextError);
progressBar.setVisibility(View.GONE);
Toast.makeText(getApplicationContext(), "Error loading ads", Toast.LENGTH_SHORT).show();
}
//Ad impression callback
@Override
public void adImpression(NativeAd nativeAd) {
super.adImpression(nativeAd);
}
});
//The native ad request object
nativeAd.loadAd(new NativeAdRequest()
// optional - config your ad request:
.setCachingPolicy(NativeAdRequest.CachingPolicy.STATIC_ONLY)
.setCreativeType(NativeAdRequest.CreativeType.ALL)
.setVideoLength(NativeAdRequest.VideoLength.SHORT)
.setVideoQuality(NativeAdRequest.VideoQuality.LOW)
);
}
private void setViews() {
nativeAdView = (NativeAdView) findViewById(R.id.na_view);
imageView = (ImageView) findViewById(R.id.na_icon);
textView = (TextView) findViewById(R.id.na_title);
mediaView = (MediaView) findViewById(R.id.na_media);
progressBar = (ProgressBar) findViewById(R.id.progressBar);
button = (Button) findViewById(R.id.install);
rating = (TextView) findViewById(R.id.rating);
description = (TextView) findViewById(R.id.description);
viewArrayList = new ArrayList < > ();
viewArrayList.add(button);
viewArrayList.add(mediaView);
}
}
Step 5: Build the view using dedicated functions
downloadAndDisplayImage(ImageView, URL string)
- This function downloads the image from a given url and put it in the target viewgetAdTitle
- This function fetches the Ad title (String, up to 25 characters)getAdDescription
- This function fetches the Ad description (String, up to 90 characters)getCTAText
- This function fetches the Ad Call-To-Action string (String)getStoreDownloads
- This function fetches the amount of downloads the advertisement's app (String)getStoreRating
- This function fetches the store rating of advertisment's app (String)
Step 6: Register the clickable areas
registerClickableViews(<array of views>)
- This function sets the clickable areas - that triggers click event. Please make sure to register only the nativeAd
views only to maintain a good UX for your user. By default, all views within the nativeAd
views are clickable, unless set specifically using the function.
Step 7: Place the Appnext Media View
We highly recommend using Appnext Media View object (com.appnext.nativeads.MediaView
) to display the ad creative.
Appnext Media View can easily show video controls buttons, the video's first slide, handle slow connections and much more.
Available functions;
setMute
- Boolean. Controls whether the video creative, when in use, play with or without sound. The default value is false.setAutoPlay
- Boolean. Controls whether the video creative, when in use, auto-play itself. The default value is true.setClickEnabled
- Boolean. Defined the functionality when the user clicks the playing video. When set to true, clicking the video will trigger click event (opens the store), when set to false, the video will pause. The default value is true.
In addition to the above functions, the MediaView
object exposes video and image Native Android functions to use
mediaView.setMute(true);
mediaView.setAutoPLay(true);
mediaView.setClickEnabled(true);
Maintain 16:9 ratio
Appnext campaigns creatives; videos, and wide images are served in 16:9 ratio. It is recommended to define the
MediaView
width and height in this ratio. By default, the creative is centered horizontally and vertically with a black background. You can change this by changing theMediaView
layout parameters.
Step 8: Configure the Appnext Privacy Policy Icon
By default, the native ads ad unit will place an "i" icon representing Appnext Ads privacy policy. Clicking it will open the Appnext Privacy Policy landing page on the device browser
The following functions are available to control the position, visibility, and color of the icon:
nativeAd.setPrivacyPolicyPosition
- Controls on which corner of thenativeAd
view the icon will show. Possibe values:PrivacyIcon.PP_ICON_POSITION_TOP_RIGHT
- Icon is located in the top-right corner of the view (Default)PrivacyIcon.PP_ICON_POSITION_TOP_LEFT
- Icon is located in the top-left corner of the viewPrivacyIcon.PP_ICON_POSITION_BOTTOM_RIGHT
- Icon is located in the bottom-right corner of the viewPrivacyIcon.PP_ICON_POSITION_BOTTOM_LEFT
- Icon is located in the bottom-left corner of the view
nativeAd.setPrivacyPolicyColor
- Controls the color texture of the "i" iconPrivacyIcon.PP_ICON_COLOR_DARK
- The icon is shown with a dark texturePrivacyIcon.PP_ICON_COLOR_LIGHT
- The icon is shown with a light texture (Default)
In order to show or hide the privacy icon -
- nativeAd.setPrivacyPolicyVisibility(PrivacyIcon.PP_ICON_VISIBILE);
- nativeAd.setPrivacyPolicyVisibility(PrivacyIcon.PP_ICON_HIDDEN);
Step 9: Configure your ad request parameters
new NativeAdRequest()
.setPostback("")
.setCategories("")
.setSpecificCategories("")
.setCachingPolicy(CachingPolicy.ALL)
/* You set the creative caching policy:
CachingPolicy.ALL - video and wide image
CachingPolicy.NOTHING - video and wide image will not be cached
CachingPolicy.VIDEO_ONLY - only the video will be cached
CachingPolicy.STATIC_ONLY - only the wide image will be cached
assets that were not cached will be streamed */
.setCreativeType(NativeAdRequest.CreativeType.ALL)
/* You filter the ad content based on your preferred creative:
CreativeType.ALL - both video and static
CreativeType.VIDEO_ONLY - video type creatives only
CreativeType.STATIC_ONLY - wide (static) creatives only */
.setVideoLength(NativeAdRequest.VideoLength.SHORT)
.setVideoQuality(NativeAdRequest.VideoQuality.HIGH)
);
By default, the native ad settings will be loaded with pre-defined settings. You can change these settings by defining the NativeAdRequest
object
setCategories
- Set preferred ad categoriessetSpecificCategories
- Set specific ad categoriessetPostback
- Postback parameters that will be posted to your server after the user installed an app (make sure to encode the values)setCachingPolicy
- Appnext supports four caching options when loading the native ad;CachingPolicy.ALL
- The video and wide image will be cached before showing the ad (Default)CachingPolicy.NOTHING
- No caching at allCachingPolicy.VIDEO_ONLY
- Only the video in the creative will be cachedCachingPolicy.STATIC_ONLY
- Only the wide image will be cached. None cached assets will be streamed
setCreativeType
- The native ad can filter the ad content based on this setting;CreativeType.ALL
- Both video and static (Default)CreativeType.VIDEO_ONLY
- Ads with video type creative onlyCreativeType.STATIC_ONLY
- Ads with static type creative only
setVideoLength
- Sets the preferred length of the ad video creativeVideoLength.SHORT
- Up to 15 seconds long videos (Default)VideoLength.LONG
- 20-60 seconds long videos
setVideoQuality
- Sets the preferred quality of the ad video creativeVideoQuality.HIGH
- Video will load in a high qualityVideoQuality.LOW
- Video will load in a low quality (Default)
Note:
- The SDK will log the impression and handle the click automatically.
You must register the ad's view with the NativeAd instance and make sure you don’t open the market using the Android package.
Step 10: Finalize Appnext
Add the following code when you destroy your Activity
@Override
public void onDestroy() {
super.onDestroy();
nativeAd.destroy();
}
Working with the ad metadata directly
We highly recommend working with the MediaView and the dedicated functions. If you require working directly with the ad creative metadata, the following getters are also available to use, including additional getters to fetch the country, supported versions, estimated ecpm and ppr values
String bannerId = nativeAd.getBannerID();
String imageURL = nativeAd.getIconURL();
String imageURLWide = nativeAd.getWideImageURL();
String urlVideo = nativeAd.getVideoUrl();
String country = nativeAd.getCountry();
String supportedVersion = nativeAd.getSupportedVersion();
float ecpm = nativeAd.getECPM();
float ppr = nativeAd.getPPR();
Example Project
You can find code examples of our Native Ads SDK here.
App Categories
1. Action 2. Adventure 3. Arcade 4. Arcade & Action 5. Board 6. Books & Reference 7. Brain & Puzzle 8. Business 9. Card 10. Cards & Casino 11. Casino 12. Casual 13. Comics 14. Communications 15. Education 16. Educational 17. Entertainment 18. Family 19. Finance 20. Health & Fitness 21. Libraries & Demo 22. Lifestyle 23. Live Wallpaper | 24. Media & Video 25. Medical 26. Music 27. Music & Audio 28. News & Magazines 29. Personalization 30. Photography 31. Productivity 32. Puzzle 33. Racing 34. Role Playing 35. Shopping 36. Simulation 37. Social 38. Sports 39. Sports Games 40. Strategy 41. Tools 42. Travel & Local 43. Trivia 44. Weather 45. Word |
Make sure to encode (%20) categories with more than 1 word, example: Travel%20%26%20Local
Advanced Configuration (Optional)
You can call for Ads based on a package name - For example, if you will call with the package name "com.whatsapp" - you will receive a campaign for WhatsApp (if available) and more Ads related to category Communication.
Request a single or multiple Ads
you can choose between
single request : requesting one native Ad or
multiple requests : return X amount of Ads at the same time. Please replace <NUMBER_OF_REQUESTS> with the numbers of Ads you would like to receive.
Single request:
NativeAd nativeAd = new NativeAd(this, "<YOUR_PLACEMENT_ID>");
nativeAd.setAdListener(new NativeAdListener() {
@Override
public void onAdLoaded(NativeAd nativeAd, AppnextAdCreativeType creativeType) {
super.onAdLoaded(nativeAd, creativeType);
}
.
.
.
});
nativeAd.getAdByPackage(new NativeAdRequest(), "<package_name>");
Multiplerequest:
AdLoader.getAdsByPackage(this, "<YOUR_PLACEMENT_ID>", "<package_name>",
new NativeAdRequest(), new NativeAdListener() {
@Override
public void onAdLoaded(NativeAd nativeAd, AppnextAdCreativeType creativeType) {
super.onAdLoaded(nativeAd, creativeType);
nativeAds.add(nativeAd);
}
.
.
.
}, <NUMBER_OF_REQUESTS>);
Integration Support
Should you have any problems integrating the product, log a ticket with us by emailing [email protected].
Updated almost 4 years ago