Banners
Appnext iOS SDK - Banners ad unit
Ad Unit Integration
Make sure to complete the Getting Started with the iOS SDK steps before you begin
Step 1: Add import statement
Add the following import statement in your pre-compiled header or in your .m/.h file where you intend to instantiate and use the Appnext SDK. If your application is written in swift add it to your bridging-header.
#import <AppnextLib/AppnextLib.h>
Step 2: Define a new Banner
Build a new BannerRequest
BannerRequest* bannerRequest = [[BannerRequest alloc] init];
bannerRequest.bannerType = LargeBanner;
Available sizes for the bannerType
Banner Type | Banner Size |
---|---|
Banner | 320x50 |
LargeBanner | 320x100 |
MediumRectangle | 300x250 |
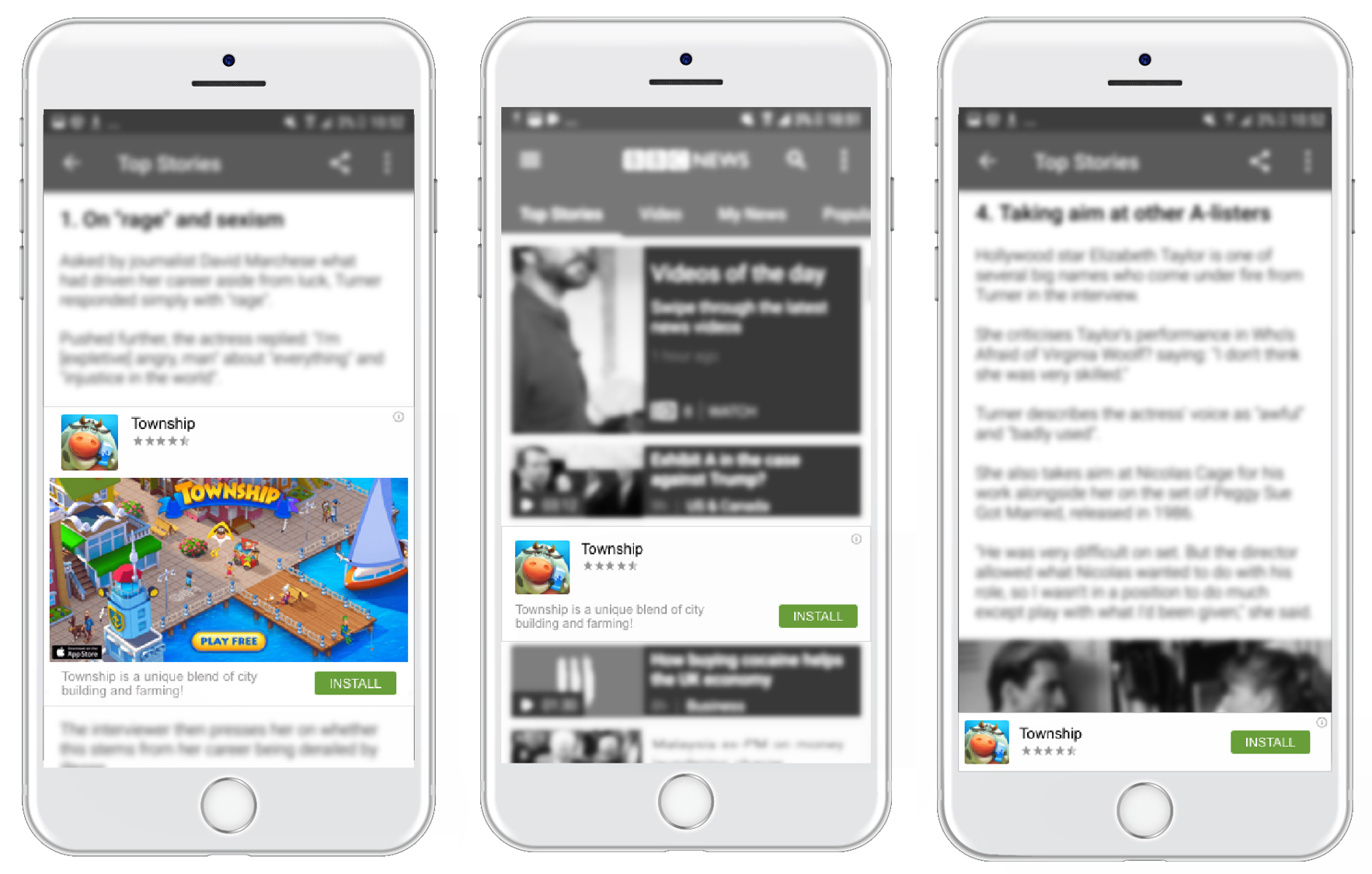
Banner sizes
Step 3: Create a new AppnextBannerView with the BannerRequest
Make sure to add a valid Appnext placement id
AppnextBannerView* bannerView = [[AppnextBannerView alloc] initBannerWithPlacementID:@“VALID_APPNEXT_PLACEMENT_ID”];
Important!
There is no need to give width or height constraints to the banner. The banner dimensions set according to the given size. Appnext will carry the banner an intrinsic content size to size the view
Step 4: Add the banner to the viewController
Add the bannerView
to the viewController with position constraints.
Please note that we don't give size constraints, as the provided banner size will give the banner an intrinsic content size to size the view.
self.bannerView.translatesAutoresizingMaskIntoConstraints = NO;
[self.view addSubview: self.bannerView];
[self.view addConstraints: @[
[NSLayoutConstraint constraintWithItem: self.bannerView
attribute: NSLayo + -utAttributeBottom
relatedBy: NSLayoutRelationEqual
toItem: self.view
attribute: NSLayoutAttributeBottom
multiplier: 1
constant: 0
], [NSLayoutConstraint constraintWithItem: self.bannerView
attribute: NSLayoutAttributeCenterX
relatedBy: NSLayoutRelationEqual
toItem: self.view
attribute: NSLayoutAttributeCenterX
multiplier: 1
constant: 0
]
]];
Step 5: Load the ad
[bannerView loadAd:bannerRequest];
Important!
Please locate the banner above the fold (visible to the user) upon loading the banner. Currently, Appnext supports impression counting for above the fold located banners.
Step 6 (Optional): Delegates - callbacks
In order to receive client-side callbacks, the client can set a delegate for each Banner:
@protocol AppnextBannerDelegate <NSObject>
@optional
- (void) onAppnextBannerLoadedSuccessfully;
- (void) onAppnextBannerError:(AppnextError) error;
- (void) onAppnextBannerClicked;
- (void) onAppnextBannerImpressionReported;
@end
Possible errors returned to client in AppnextBannerDelegate
method onAppnextBannerError:error
:
typedef NS_ENUM(NSInteger, AppnextError) {
AppnextNoAds,
AppnextAdNotReady,
AppnextConnectionError,
AppnextNoMarket,
AppnextTimeOut
};
Configuring the Appnext Banners
Custom Configuration
By default, the Banner configuration will be loaded with default values. You can change the configuration directly by property or through the setter function within the BannerRequest
BannerRequest* bannerRequest = [[BannerRequest alloc] init];
Example
//320x50 and 320x100:
BannerRequest * bannerRequest = [
[BannerRequest alloc] init];
[bannerRequest setCategories: [NSArray arrayWithObjects: @ @ "category1", @ "category2", nil]];
[bannerRequest setPostback: @ "postback"];
[bannerRequest setBannerType: LargeBanner]; // default is Banner
//300x250:
BannerRequest * bannerRequest = [
[BannerRequest alloc] init];
[bannerRequest setCategories: [NSArray arrayWithObjects: @ @ "category1", @ "category2", nil]];
[bannerRequest setPostback: @ "postback"];
[bannerRequest setBannerType: LargeBanner]; // default is Banner
BannerRequest * bannerRequest = [
[BannerRequest alloc] init];
[bannerRequest setCategories: [NSArray arrayWithObjects: @ @ "category1", @ "category2", nil]];
[bannerRequest setPostback: @ "postback"];
[bannerRequest setCreative: Static]; // default is Static
[bannerRequest setAutoPlay: YES]; // default is NO
[bannerRequest setMute: YES]; // default is YES
[bannerRequest setVideoLength: Short]; // default is Auto
[bannerRequest setClickEnabled: YES]; // default is YES
[bannerRequest setClickInApp: YES]; // default is YES
List of configuration options
By default, the banner settings will be loaded with pre-defined settings. You can change these settings by defining the BannerRequest
object
Setters that are relevant to all banner sizes:
setCategories
- String. Set preferred ad categoriessetPostback
- String. Postback parameters that will be posted to your server after the user installed an app (make sure to encode the values)setClickInApp
- Determines the App Store loading method, when set to "YES" - the App Store will open "in-app" within the running app, Otherwise the App Store will open outside the running app
Setters that are relevant only to the MediumRectangle
banner size:
setCreative
- TheMediumRectangle
size can show video in addition to static creative. When video is in use, the file will be streamed (default value isALL
- managed by Appnext)Static
Video
All
setAutoPlay
- Boolean. When a video is set as the creative, this setter defining if the video will auto play or not (default value isNO
)setMute
- Boolean. When a video is set as the creative, this setter is defining if the video will start on muted volume or not. Un-mute/Mute button is located on the video creative. (default value isYES
- the video is muted)setVideoLength
- When a video is set as the creative, this setter is defining the preferred length of the video. (default value isAUTO
)Short
- Up to 15 seconds long videosLong
- 20-60 seconds long videos
setClickEnabled
- Boolean. Defined the functionality when the user clicks the playing video. When set toYES
, clicking the video will trigger click event (opens the store), when set to false, the video will pause. The default value isYES
.
Example Project
Please find example project BannersTest
or how to implement the Banners ad unit inside the zip file here.
App Categories
1. Action 2. Adventure 3. Arcade 4. Board 5. Books 6. Business 7, Card 8. Casino 9. Catalogs 10. Dice 11. Education 12. Educational 13. Entertainment 14. Family 15. Finance 16. Food & Drink 17. Graphics & Design 18. Health & Fitness 19. Kids | 20. Lifestyle 21. Medical 22. Music 23. Navigation 24. News 25. Photo & Video 26. Productivity 27. Puzzle 28. Racing 29. Reference 30. Role Playing 31. Simulation 32. Social Networking 33. Sports 34. Strategy 35. Travel 36. Trivia 37. Utilities 38. Weather |
Integration Support
Should you have any problems integrating the product, log a ticket with us by emailing [email protected].
Updated 10 months ago